Kodeclik Blog
Python’s If Not Operator
Python’s if not operator tests if some condition is not true and can be a succinct way to describe some types of program logic. It is useful when a variable records a certain condition but the conditional tests for the negation of that condition.
Here is a simple example of Python’s if not operator:
is_it_raining = False
if (not is_it_raining):
print("We can play Tennis!")
This results in:
We can play Tennis!
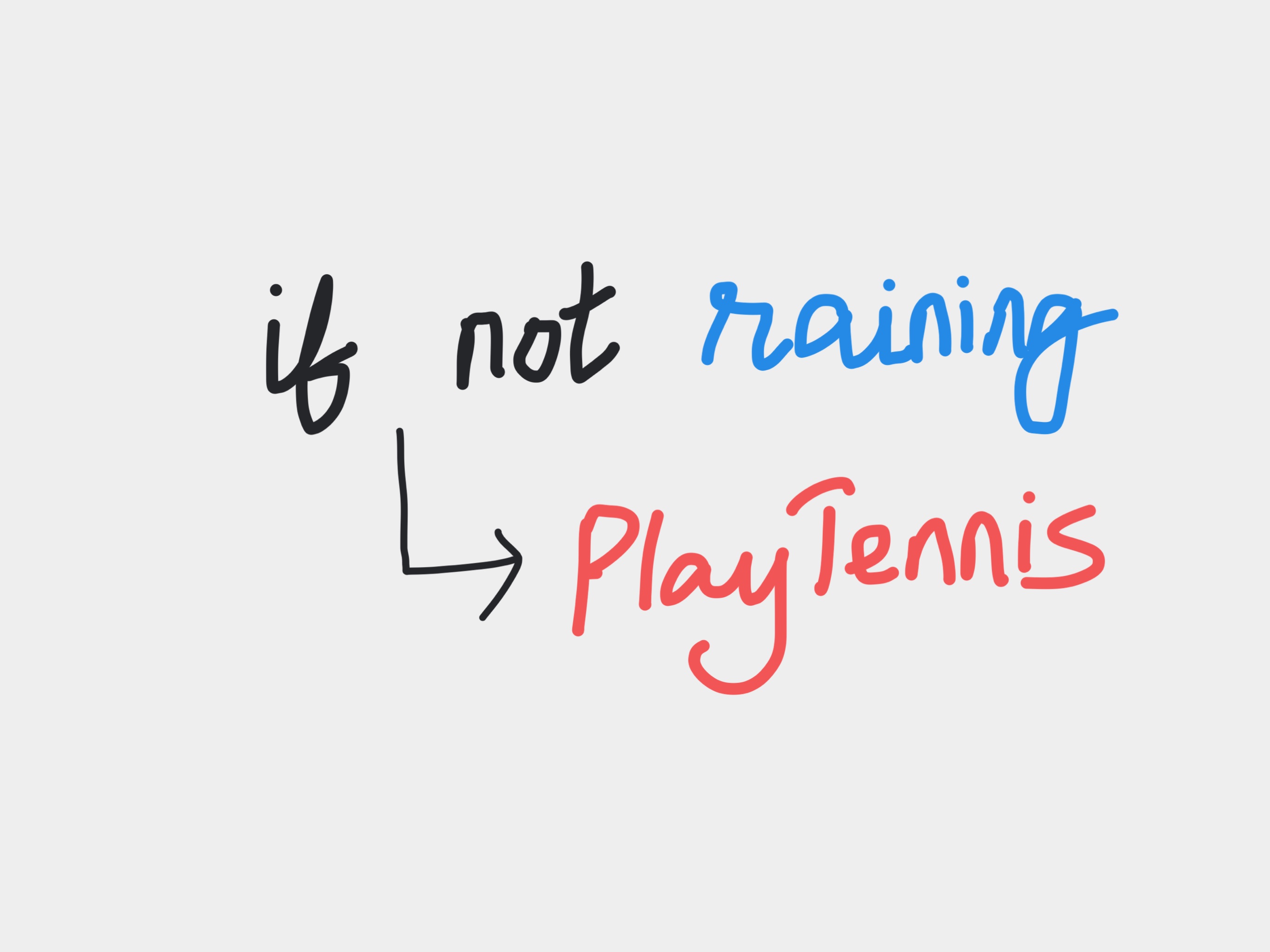
if not with lists or strings
The expression following the not need not be boolean. It can be any list or string. Note that an empty list or empty string will evaluate to False and any other value will evaluate to be True.
Consider the following program:
customer_name = "Mickey Mouse"
if (not customer_name):
print("Customer Name not found")
else:
print("Customer Name is available")
This outputs:
Customer Name is available
On the other hand, with the below program:
customer_name = ""
if (not customer_name):
print("Customer Name not found")
else:
print("Customer Name is available")
we will get:
Customer Name not found
The same logic applies to lists:
mylist = []
yourlist = ['a',1,2]
if (not mylist):
print("mylist is empty")
else:
print("mylist is not empty")
will give:
mylist is empty
On the other hand, with the below program:
mylist = []
yourlist = ['a',1,2]
if (not yourlist):
print("yourlist is empty")
else:
print("yourlist is not empty")
we get:
yourlist is not empty
Similar to strings and lists, the if not can be used with other iterables like dictionaries and tuples. In all these cases, it makes for more readable code.
Interested in more things Python? See our blogpost on Python's enumerate() capability. Also if you like Python+math content, see our blogpost on Magic Squares. Finally, master the Python print function!
Want to learn Python with us? Sign up for 1:1 or small group classes.