Kodeclik Blog
What is NaN in Javascript?
We will learn what NaN (Not a Number) means in Javascript. For this purpose, we will write a Javascript program and embed it inside a HTML page like so:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Nan in Javascript</title>
</head>
<body>
<script>
</script>
</body>
</html>
In the above HTML page you see the basic elements of the page like the head element containing the title (“Nan in Javascript”) and a body with an empty script tag. Any HTML markup goes inside the body tags and any Javascript code goes inside the script tags.
NaNs are the result of nonsensical operations
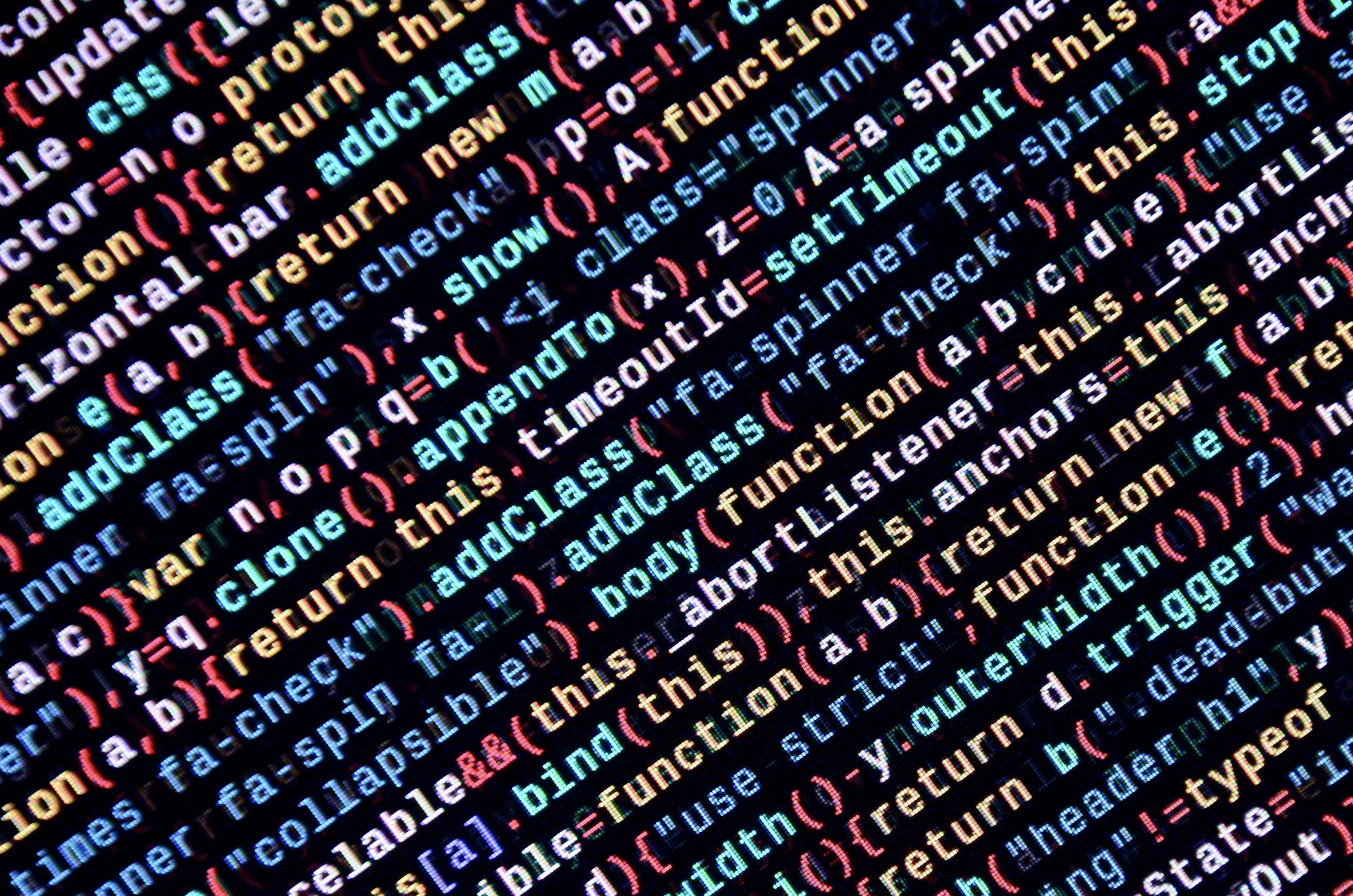
Consider the following code that attempts to multiply a string by an integer.
<script>
const n = "Kodeclik" * 10
document.write("Kodeclik multiplied by 10 is: " + n)
</script>
The output is:
Kodeclik multiplied by 10 is: NaN
Any similar nonsensical operation will also lead to an NaN.
Another example of an NaN is if we try to find square roots of negative numbers:
<script>
const n = Math.sqrt(-1)
document.write("square root of -1 is: " + n)
</script>
The output is:
square root of -1 is: NaN
NaNs used in operations will lead to more NaNs
Consider the following code:
<script>
const n = NaN * 10
document.write("NaN multiplied by 10 is: " + n)
</script>
The output is:
NaN multiplied by 10 is: NaN
If we try to find the square root of a NaN, we will again get an NaN:
<script>
const n = Math.sqrt(NaN)
document.write("NaN's square root is: " + n)
</script>
with the output:
NaN's square root is: NaN
Division by zero
Let us attempt to divide an integer by zero:
<script>
const n = 6/0;
document.write("6 divided by zero is: " + n)
</script>
The output is:
6 divided by zero is: Infinity
So it appears like Javascript has a literal called Infinity. If you attempt to multiply Infinity by, say, zero:
<script>
const n = 0*(6/0);
document.write("0 times (6 divided by zero) is: " + n)
</script>
we obtain:
0 times (6 divided by zero) is: NaN
So infinity times zero is a NaN. Infinity divided by infinity is also an NaN:
<script>
const n = 6/0
document.write(n + " divided by " + n + " is " + n/n)
</script>
The output is:
Infinity divided by Infinity is NaN
Checking if an object is a NaN
The predicate isNaN() returns true if its input is not a number and false otherwise. Here is an example of how to use it:
<script>
function sanitize(n) {
if (isNaN(n)) {
document.write(n + " is not a number<BR>")
} else {
document.write(n + " is a proper number<BR>")
}
}
sanitize(3)
sanitize(6/0)
sanitize("hello")
</script>
The output is:
3 is a proper number
Infinity is a proper number
hello is not a number
As expected, 3 is not an NaN and Infinity is also not an NaN (as we saw in the example earlier). Of the three inputs, only “hello” is an NaN.
Assigning NaN values to variables
Sometimes you will have a need to assign NaN explicitly to variables.
<script>
const x = NaN
document.write("x's value is: " + x + "<BR>")
</script>
The output is:
x's value is: NaN
You can use this capability when you want to keep track of illegal values for variables in your program. For instance if you are requesting a customer for their age in a program (intending to later doing some operations with it) and the customer enters a string or non-numeric for their age, you can assign a NaN to it and then future operations will be able to detect that they have a not-a-number for the age.
NaNs are not equal to NaNs
Because NaN simply denotes “not a number” two variables that have values as NaN are not necessarily equal to each other. Consider the following program:
<script>
const x = NaN
const y = NaN
if (x == y) {
document.write(x + " is equal to " + y)
} else {
document.write(x + " is not equal to " + y)
}
</script>
The output is:
NaN is not equal to NaN
Note that this is true irrespective of whether you use “==” or “===” in your if condition.
In fact, this property of NaNs (i.e., it is not equal to itself) can be used as a check to see if a given input is an NaN (simply check if it is equal to itself). This gives you an alternative to using the isNaN() function we encountered earlier.
If you liked learning about NaNs, checkout our blogpost on computing powers and how NaNs occur in those operations. Another case where NaNs occur is when you are processing user input. See our detailed blogpost on gathering user input.
Interested in learning more Javascript? Learn how to loop through two Javascript arrays in coordination and how to make a Javascript function return multiple values! Also learn about Javascript's 'if not' construct.
Want to learn Javascript with us? Sign up for 1:1 or small group classes.